Eclipse software is, like, the OG IDE for so many devs. It’s super versatile, thanks to its massive plugin ecosystem, meaning you can totally customize it for whatever you’re working on – Java, Python, C++, you name it. This guide dives into everything Eclipse, from setting up your first project to mastering debugging and even tackling those pesky plugin conflicts.
Table of Contents
Get ready to level up your coding game!
We’ll cover the basics of the Eclipse IDE, showing you how to navigate its interface and leverage its core functionalities. Then we’ll jump into more advanced topics, like managing large projects, collaborating with teammates using Git, and troubleshooting common issues. We’ll also explore different Eclipse versions and plugins, comparing their features and helping you choose the best tools for your needs.
Think of this as your ultimate Eclipse survival guide!
Eclipse IDE Overview
Eclipse is a wildly popular, open-source Integrated Development Environment (IDE) known for its flexibility and extensive plugin ecosystem. It’s used by developers across a wide range of programming languages and platforms, making it a versatile tool for building everything from simple applications to complex enterprise systems. Its core strength lies in its modular architecture, allowing for customization and extension to suit individual needs.Eclipse’s core functionalities center around code editing, debugging, and project management.
The editor provides features like syntax highlighting, code completion, and refactoring tools that significantly boost developer productivity. The debugger allows developers to step through code, inspect variables, and identify errors. Project management features facilitate organizing code, managing dependencies, and building applications. Beyond these basics, Eclipse’s true power lies in its extensibility.
Eclipse Platform Architecture
The Eclipse platform is built upon a layered architecture. At its base is the Platform Runtime, providing core services like the operating system abstraction layer, windowing system, and the plugin framework. Above this sits the workbench, which provides the user interface, including views, editors, and perspectives. These perspectives allow developers to tailor their workspace to specific tasks, such as Java development or web development.
Finally, the top layer consists of various plugins that provide specific functionalities, like support for different programming languages or debugging tools. This modular design allows for seamless integration of new features without affecting the core functionality. For example, a plugin for C++ development can be added without impacting the functionality of a Java plugin.
The Eclipse Plugin Ecosystem
The plugin ecosystem is arguably Eclipse’s most significant asset. It allows developers to extend the IDE’s capabilities far beyond its core functionalities. Thousands of plugins are available, covering a vast range of programming languages (Java, C++, Python, PHP, and many more), frameworks (Spring, Hibernate, React), and tools (version control integration, testing frameworks, and database management tools). This vast library allows developers to customize their Eclipse environment to perfectly match their project requirements.
For instance, a developer working on a Java web application might install plugins for Spring, Maven, and a web server integration, creating a tailored development environment. The availability of these plugins drastically reduces development time and improves efficiency by providing ready-made tools and integrations. This constant evolution and expansion driven by the community ensures Eclipse remains relevant and adaptable to the ever-changing landscape of software development.
Eclipse Software Development
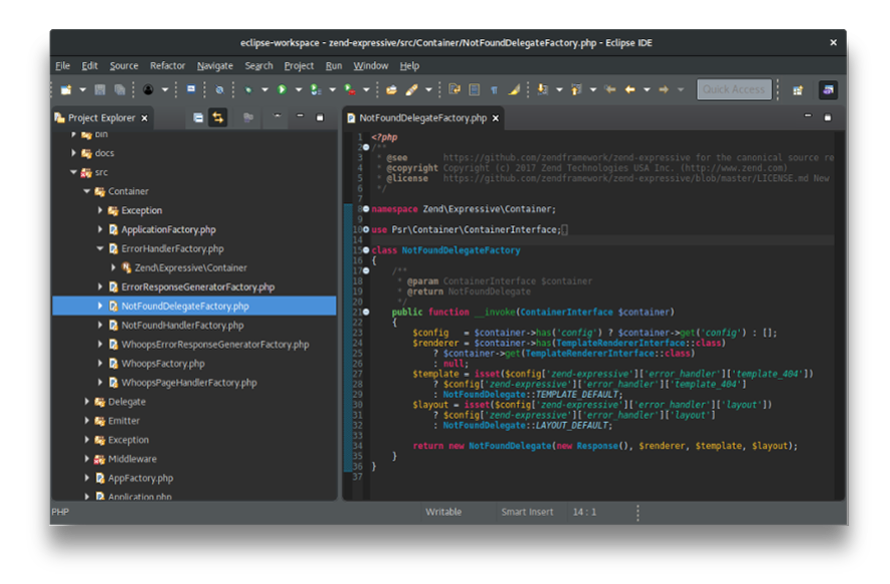
Okay, so you’ve got a handle on the Eclipse IDE itself – now let’s dive into actuallyusing* it to build stuff. This section will walk you through creating a simple Java application, managing your projects effectively, and mastering the art of debugging within Eclipse. Think of this as your crash course in Eclipse-powered Java development.
Creating a Simple Java Application
Creating a basic Java “Hello, World!” program in Eclipse is straightforward. First, you’ll create a new Java project. Then, you’ll add a new class to that project. Finally, you’ll write the code, run it, and bask in the glory of your first Eclipse-built application. Let’s break it down step-by-step:
- Create a New Java Project: Go to File > New > Java Project. Give your project a name (e.g., “HelloWorld”). Make sure the correct JRE (Java Runtime Environment) is selected. Click Finish.
- Create a New Class: Right-click on your project in the Project Explorer, select New > Class. Give your class a name (e.g., “Main”). Check the box that says “public static void main(String[] args)”. Click Finish.
- Write the Code: Eclipse will generate a basic class structure. Inside the `main` method, add the following line:
System.out.println("Hello, World!");
- Run the Application: Right-click anywhere within the `Main.java` file and select Run As > Java Application. You should see “Hello, World!” printed in the Console view.
Managing Projects in Eclipse
Effective project management is key to avoiding a tangled mess of code. Eclipse offers several features to keep your projects organized. Good organization means easier collaboration, quicker debugging, and less hair-pulling frustration.
- Use a Consistent Project Structure: Maintain a logical directory structure within your project. Group related files together (e.g., all classes related to a specific feature in a single package).
- Leverage Package Organization: Use packages to organize your classes logically. This prevents naming conflicts and improves code readability. Think of packages as folders for your classes.
- Employ Version Control (e.g., Git): Integrate a version control system like Git to track changes, collaborate with others, and easily revert to previous versions if needed. Eclipse has excellent Git integration.
- Regularly Clean and Build: Periodically clean your project to remove unnecessary files and rebuild it to ensure everything is up-to-date and free of errors. This helps prevent unexpected issues down the line.
Debugging Java Code with Eclipse
Debugging is an essential skill for any programmer. Eclipse’s debugger is a powerful tool that allows you to step through your code line by line, inspect variables, and identify the source of errors. Mastering the debugger will save you countless hours of frustration.
- Set Breakpoints: Click in the left margin next to the line numbers to set breakpoints. Execution will pause at these points.
- Start Debugging: Right-click within your code and select Debug As > Java Application. The debugger will start, pausing at your breakpoints.
- Step Through Code: Use the step over (F6), step into (F5), and step return (F7) buttons to move through your code. Step over executes the next line without stepping into methods. Step into enters a method call, and step return exits the current method.
- Inspect Variables: Use the Variables view to inspect the values of variables at different points in your code. This helps you understand the flow of data and identify issues.
- Use the Expressions View: The Expressions view lets you evaluate expressions in real-time while debugging. This is handy for checking complex calculations or conditions.
Eclipse Versions and Updates
Keeping your Eclipse IDE up-to-date is crucial for accessing the latest features, performance improvements, and security patches. Different versions offer varying functionalities and performance characteristics, so understanding the update process and potential compatibility issues is key to a smooth development experience.Choosing the right Eclipse version depends on your project needs and system resources. While newer versions often boast improved performance and new features, they might also introduce unexpected compatibility problems with existing plugins or projects.
Eclipse Version Comparison: 2023-09 vs. 2022-12
The differences between consecutive Eclipse releases, such as 2023-09 and 2022-12, are usually incremental. You might find enhancements in the Java editor, improved debugging tools, or updated support for newer Java versions. Performance improvements are often subtle but noticeable, especially with larger projects. For example, 2023-09 might offer faster startup times and improved responsiveness compared to 2022-12, but the exact degree of improvement varies based on hardware and project complexity.
Specific feature changes are usually documented in the release notes for each version, available on the Eclipse website. Checking these notes before upgrading is highly recommended.
Updating Eclipse to the Latest Version
Updating Eclipse is generally straightforward. The process typically involves downloading the latest version from the official Eclipse website and then using the existing Eclipse installation to import your workspace and settings. Many Eclipse distributions include an automatic update mechanism within the IDE itself. This usually involves checking for updates through the “Help” menu, and then following the on-screen prompts to download and install the newer version.
Eclipse will generally attempt to preserve your existing settings and plugins, but manual verification after the update is prudent. Back up your workspace before performing any major update to ensure you can restore your projects if problems arise.
Potential Compatibility Issues and Mitigation Strategies
Upgrading Eclipse can sometimes lead to compatibility issues. Older plugins might not be compatible with the newer version, leading to errors or unexpected behavior. Similarly, projects using outdated libraries or frameworks might experience build failures. Before updating, review the release notes for any known compatibility issues or breaking changes. If you use many plugins, consider updating them individuallybefore* upgrading the main Eclipse IDE.
This allows you to identify and resolve plugin conflicts early. If compatibility problems arise after the upgrade, carefully review error messages and consult the documentation for both the IDE and the affected plugins. Reverting to a previous version might be necessary in extreme cases, but backing up your workspace before any update minimizes the impact of such a step.
Eclipse Plugins and Extensions
Eclipse’s power lies not just in its core functionality, but in its vast ecosystem of plugins and extensions. These add-ons allow you to tailor the IDE to your specific needs, boosting productivity and streamlining your workflow. Whether you’re a seasoned Java developer or just starting out, exploring the plugin marketplace is a crucial step in mastering Eclipse.
Plugins extend Eclipse’s capabilities by adding new features, integrating with other tools, or enhancing existing ones. They range from simple code formatting tools to complex frameworks for specific development tasks. Choosing the right plugins can significantly improve your development experience, making coding faster, more efficient, and more enjoyable. Conversely, poorly chosen or poorly maintained plugins can lead to conflicts, instability, and frustration.
Careful consideration is key.
Essential Plugins for Java Development
Several plugins are considered practically essential for serious Java development within Eclipse. These tools enhance core functionality, providing features that significantly improve developer productivity and code quality. The selection below represents a solid starting point, though individual needs may vary.
- Eclipse Java Development Tools (JDT): This is the core plugin for Java development in Eclipse, providing essential features like code completion, debugging, and refactoring.
- Maven Integration for Eclipse (m2e): Simplifies the process of building and managing Java projects using Apache Maven, a popular build automation tool.
- EGit: Provides seamless integration with Git, allowing you to manage your code repositories directly within Eclipse.
- FindBugs: A static analysis tool that helps identify potential bugs and vulnerabilities in your Java code.
- Checkstyle: Enforces coding style conventions, improving code readability and maintainability.
Advantages and Disadvantages of Popular Eclipse Plugins
The decision to use a specific plugin involves weighing its benefits against potential drawbacks. While many plugins offer substantial advantages, it’s crucial to understand potential limitations or compatibility issues before installation.
For example, a plugin offering advanced refactoring capabilities might come with a steeper learning curve, requiring time investment to master its features. Conversely, a simpler plugin might lack the advanced functionalities offered by more complex alternatives. Understanding these trade-offs is crucial for making informed decisions.
Comparison of Popular Plugins
This table compares four popular Eclipse plugins, highlighting their key features and use cases. Note that plugin functionality and user experience can change with updates, so always refer to the latest documentation.
Plugin Name | Key Features | Use Cases | Advantages/Disadvantages |
---|---|---|---|
m2e (Maven Integration) | Maven project management, dependency resolution, build execution | Building and managing Java projects using Maven | Advantage: Streamlines Maven workflow. Disadvantage: Can be complex for beginners. |
EGit (Git Integration) | Git repository management, branching, merging, commit history | Version control within Eclipse | Advantage: Convenient Git access. Disadvantage: Can be resource-intensive for large repositories. |
FindBugs | Static code analysis, bug detection, vulnerability identification | Improving code quality and identifying potential issues | Advantage: Proactive bug detection. Disadvantage: Can generate false positives. |
Checkstyle | Code style enforcement, automated code formatting | Maintaining consistent coding style across projects | Advantage: Improved code readability and maintainability. Disadvantage: Requires configuration and can be strict. |
Eclipse for Different Programming Languages
Eclipse, while initially known for its Java prowess, is remarkably versatile. Its extensible architecture allows it to support a wide array of programming languages through the use of plugins. This adaptability makes Eclipse a powerful and consistent IDE across different development projects, regardless of the language used. This section will delve into configuring Eclipse for C++ and Python development, highlighting key differences in project setup and build processes compared to Java.
Configuring Eclipse for C++ Development
Setting up Eclipse for C++ development involves installing the necessary CDT (C/C++ Development Tooling) plugin. This plugin provides the essential tools for writing, compiling, debugging, and running C++ code within the Eclipse environment. After installing Eclipse (ideally the standard edition, as it allows for broader plugin support), you’ll need to navigate to the Eclipse Marketplace (Help > Eclipse Marketplace…).
Search for “CDT” and install the latest version of the C/C++ Development Tooling. Once installed, you can create a new C++ project by selecting “C++ Project” from the “New” menu. You’ll then specify project details such as project name, project type (e.g., Executable, Shared Library), and toolchain settings (compiler, linker, etc.). The CDT plugin integrates seamlessly with common build systems like Make and provides features such as code completion, syntax highlighting, and debugging capabilities specifically tailored for C++.
Setting up Eclipse for Python Development
To develop Python applications in Eclipse, you’ll need to install the PyDev plugin. Similar to the CDT for C++, PyDev provides Python-specific features like intelligent code completion, debugging, and integration with various Python interpreters. To install PyDev, go to the Eclipse Marketplace (Help > Eclipse Marketplace…) and search for “PyDev”. Install the plugin and restart Eclipse. After installation, create a new PyDev project.
You’ll be prompted to specify an interpreter, which is the path to your Python installation. This is crucial as PyDev uses the specified interpreter for code execution and analysis. PyDev also offers features like code refactoring, unit testing support, and integration with popular Python frameworks and libraries.
Project Setup and Build Processes: Java, C++, and Python
The project setup and build processes differ significantly across Java, C++, and Python projects within Eclipse. Java projects typically utilize the built-in Java Development Tools (JDT) and leverage the project’s classpath for managing dependencies. Building a Java project usually involves compiling the source code into bytecode and packaging it into JAR files. C++ projects, on the other hand, often rely on external build systems like Make or CMake.
These systems manage the compilation process, linking of libraries, and generation of executables. The CDT plugin integrates with these build systems, providing convenient ways to build and debug C++ projects within Eclipse. Python projects, thanks to PyDev, offer a more streamlined approach. Projects are often organized simply by source code files and modules. PyDev allows for direct execution of Python scripts without a complex build process.
While PyDev offers options for creating executables, it’s less frequently needed compared to the formal build processes of Java and C++ projects.
Eclipse and Version Control Systems

Version control is absolutely crucial for any software project, especially collaborative ones. It allows developers to track changes, revert to previous versions, and work together on the same codebase without overwriting each other’s work. Eclipse integrates seamlessly with several popular version control systems (VCS), most notably Git and Subversion (SVN). This section will focus on how to leverage these tools within the Eclipse environment for efficient software development.Integrating a VCS into your workflow improves code quality, reduces conflicts, and streamlines the development process.
Understanding the basics of how Eclipse handles these systems is essential for any serious programmer.
Integrating Eclipse with Git
To get started with Git in Eclipse, you’ll first need to make sure you have Git installed on your system. Once installed, you can easily integrate it with Eclipse. Here’s a step-by-step guide:
- Install the EGit plugin: In Eclipse, go to Help > Eclipse Marketplace…. Search for “EGit” and install the Eclipse Git Team Provider. This plugin provides the necessary tools for interacting with Git repositories within Eclipse.
- Create a Git repository: Right-click on your project in the Project Explorer, select Team > Share Project…. Choose Git and follow the prompts to create a local Git repository for your project. This will initialize a .git folder within your project directory, tracking all changes.
- Commit changes: After making changes to your code, right-click on your project or specific files, select Team > Commit…. This opens a window where you can review your changes and write a commit message describing the modifications. This is crucial for tracking your work and collaborating with others.
- Push changes to a remote repository: Once you’ve committed your changes locally, you can push them to a remote repository (like GitHub, GitLab, or Bitbucket). Right-click on your project, select Team > Remote > Push…. You’ll need to provide the URL of your remote repository and your credentials.
- Pull changes from a remote repository: To get the latest changes from a remote repository, right-click on your project and select Team > Remote > Pull…. This will download the latest code and merge it into your local copy. Be prepared to resolve any potential merge conflicts.
Best Practices for Using Git within Eclipse for Collaborative Development
Effective collaboration with Git requires adherence to certain best practices. These practices enhance teamwork and minimize conflicts.
- Use descriptive commit messages: Clearly explain the purpose and scope of each commit. This helps others (and your future self) understand the changes made.
- Commit frequently: Make small, focused commits rather than large, monolithic ones. This simplifies the process of reviewing changes and makes it easier to revert to earlier versions if necessary.
- Branch effectively: Use branches to isolate new features or bug fixes from the main development line. This prevents instability in the main codebase and allows for parallel development.
- Resolve merge conflicts promptly: When merge conflicts occur, address them promptly and carefully. Eclipse provides tools to help you resolve these conflicts visually.
- Regularly push and pull changes: Keep your local and remote repositories synchronized to avoid significant divergence and potential integration problems.
Git and SVN Comparison within the Eclipse IDE
Both Git and SVN are popular version control systems, but they differ significantly in their architecture and functionality. Eclipse supports both, offering similar integration features but with distinct workflows.
Feature | Git | SVN |
---|---|---|
Architecture | Distributed (each developer has a full copy of the repository) | Centralized (single central repository) |
Branching | Lightweight and efficient branching | Branching can be more complex and resource-intensive |
Offline Work | Full offline capabilities | Limited offline capabilities |
Collaboration | Facilitates collaborative workflows through branching and merging | Collaboration is primarily managed through the central repository |
Learning Curve | Steeper initial learning curve, but more powerful in the long run | Generally easier to learn initially |
Eclipse and Team Development

Eclipse, beyond its individual coding prowess, shines as a collaborative powerhouse for team-based software development. Its features facilitate efficient project management, streamlined workflows, and enhanced communication, crucial for navigating the complexities of large-scale projects. This section explores how Eclipse supports team development, focusing on its role in Agile methodologies and code review processes.Managing large-scale projects effectively necessitates a structured approach.
Eclipse’s integrated tools provide a framework for this, allowing teams to break down large projects into smaller, manageable modules. This modularity, combined with Eclipse’s robust version control system integration, enables parallel development and reduces conflicts. Features like task management plugins, integrated debuggers, and shared project repositories are vital in this context. Imagine a team of 20 developers working on a complex financial application; without a robust platform like Eclipse, coordinating code changes, resolving conflicts, and ensuring consistent builds would be a logistical nightmare.
With Eclipse, each developer can work on their assigned module, with the system managing the integration and version control.
Eclipse’s Role in Agile Development, Eclipse software
Eclipse’s flexibility aligns seamlessly with the iterative nature of Agile methodologies like Scrum and Kanban. Its support for continuous integration and continuous delivery (CI/CD) pipelines is key. Agile frameworks emphasize frequent releases and iterative development, and Eclipse’s plugins facilitate this by automating build processes, running tests, and deploying updates. For example, a team using Scrum can leverage Eclipse’s integration with Jira or other project management tools to track sprints, assign tasks, and monitor progress.
The ability to quickly iterate on code and receive feedback within the Eclipse environment directly contributes to the rapid development cycles inherent in Agile.
Facilitating Code Reviews and Collaboration
Code reviews are fundamental to software quality. Eclipse provides a strong foundation for effective code review processes through features like integrated diff viewers, which highlight changes made between versions. Many plugins enhance this functionality further, providing tools for collaborative code review, enabling developers to annotate code, leave comments, and track revisions within the Eclipse environment. Imagine a scenario where a junior developer submits a code change.
Senior developers can use Eclipse’s code review tools to inspect the code, provide feedback, and suggest improvements before merging it into the main branch. This process minimizes errors and ensures consistent coding standards. The ability to conduct these reviews directly within the IDE fosters a collaborative environment, reducing the friction often associated with external code review tools.
Troubleshooting Common Eclipse Issues
Eclipse, while a powerful IDE, isn’t immune to problems. From minor annoyances to major roadblocks, encountering issues is a part of the development process. This section will cover some common Eclipse problems and provide practical solutions to get you back on track quickly. Understanding these common pitfalls will save you valuable time and frustration.
Many Eclipse issues stem from simple configuration errors, corrupted files, or plugin conflicts. Effective troubleshooting often involves systematically checking the most likely causes before moving to more complex solutions. Remembering to save your work frequently can also prevent data loss should a problem occur.
Workspace Issues and Corrupted Projects
A corrupted workspace can manifest in various ways, including slow performance, inability to load projects, or unexpected errors. The workspace is essentially Eclipse’s central hub, storing project settings and other vital information. A corrupted workspace can render your entire development environment unusable. Regular backups are crucial to mitigating this risk.
To resolve workspace issues, the first step is often creating a new workspace. This involves launching Eclipse and selecting a new directory for the workspace. Then, you can import your projects from the old, potentially corrupted workspace into the new one. If individual projects are corrupted, you may need to delete the project folder and re-import it from version control (if available), or reconstruct the project from backups.
If the issue persists, reinstalling Eclipse might be necessary, though it’s a last resort.
Plugin Conflicts and Performance Problems
Eclipse’s extensibility through plugins is a double-edged sword. While plugins enhance functionality, conflicts between them can lead to crashes, unexpected behavior, or significant performance degradation. This often manifests as slow startup times, unresponsive interfaces, or errors related to specific plugins.
Troubleshooting plugin conflicts typically involves disabling plugins one by one to identify the culprit. This can be done through Eclipse’s Preferences (Window > Preferences > Install/Update > Installed Software). Once the problematic plugin is identified, you can either update it to a newer version (if available), uninstall it, or search for an alternative plugin with less conflict potential.
Regularly updating your plugins can often prevent many of these conflicts.
Common Errors and Their Solutions
Eclipse throws various error messages, each indicating a specific problem. Common errors include “NullPointerException,” “ClassNotFoundException,” and build path errors. These often point to problems in your code, missing libraries, or incorrect project configurations.
Addressing these errors often requires careful code review and debugging. “NullPointerExceptions” indicate that you’re trying to use a variable that hasn’t been initialized. “ClassNotFoundExceptions” suggest that a required class isn’t on the project’s classpath. Build path errors usually stem from missing dependencies or incorrect project settings. Eclipse’s debugging tools, such as breakpoints and step-through execution, are invaluable for pinpointing the source of these errors.
Eclipse’s Future and Roadmap
Eclipse, a veteran in the IDE world, isn’t resting on its laurels. The project continues to evolve, adapting to the ever-shifting landscape of software development methodologies and technologies. Its future hinges on its ability to remain a flexible, extensible platform capable of supporting the next generation of development practices.The Eclipse Foundation actively shapes the IDE’s trajectory, responding to community feedback and industry trends.
This responsiveness ensures that Eclipse remains relevant and competitive, offering developers the tools they need for current and future projects. This includes not only improvements to core functionality but also a strong emphasis on integration with emerging technologies.
Eclipse’s Focus on Cloud-Native Development
Cloud-native development is a major focus for Eclipse’s future. We’re seeing increased integration with cloud platforms like Kubernetes and advancements in tooling for microservices architecture. This involves enhanced support for containerization technologies (like Docker and Podman), improved debugging capabilities for distributed systems, and streamlined workflows for deploying and managing applications in cloud environments. For example, expect to see improved tooling for building and deploying serverless functions, making cloud development within Eclipse smoother and more intuitive.
Enhanced AI and Machine Learning Integration
The incorporation of AI and machine learning capabilities is another key area of development. Future versions of Eclipse might include intelligent code completion that goes beyond simple suggestions, offering more contextually aware and predictive assistance. This could involve features like automated bug detection, intelligent refactoring suggestions, and even automated code generation based on natural language descriptions. Imagine Eclipse anticipating your coding needs and proactively suggesting optimal solutions, significantly boosting development speed and reducing errors.
This isn’t science fiction; several IDEs are already exploring similar capabilities.
Improved Collaboration and Remote Development Features
Remote development capabilities are becoming increasingly important. Eclipse is likely to see significant improvements in its support for remote development environments, enabling developers to work seamlessly on codebases hosted on remote servers or cloud platforms. This includes enhancements to remote debugging, improved synchronization of project files, and better support for collaborative coding scenarios. Think of a scenario where multiple developers, located geographically dispersed, can seamlessly work on the same project within Eclipse, with real-time collaboration features similar to those seen in other collaborative coding platforms.
Continued Expansion of Language Support
While already supporting a vast array of programming languages, Eclipse will likely continue to broaden its language support to encompass newer and emerging languages. This might include improved support for languages gaining traction in specific domains, such as languages tailored for data science or specialized hardware. The expansion would include not just syntax highlighting and basic code completion but also advanced features like debugging and refactoring tailored to the specific language’s nuances.
This ensures Eclipse remains a versatile tool for developers working across a diverse range of technologies.
So, I’m totally swamped with this Eclipse software project, coding all day and night. To unwind, I usually listen to music, and finding the right tunes is key. That’s where a solid best youtube to mp3 converter comes in handy for grabbing tracks from YouTube. Back to Eclipse, though – gotta finish this before the deadline!
Wrap-Up: Eclipse Software

So, there you have it – a whirlwind tour of Eclipse software! From its humble beginnings as a Java IDE to its current status as a powerhouse for multiple programming languages, Eclipse continues to evolve and adapt to the ever-changing landscape of software development. Mastering Eclipse unlocks a world of possibilities, making you a more efficient and effective coder.
Whether you’re a seasoned pro or just starting out, hopefully, this guide has given you the tools and knowledge to conquer the world of Eclipse and beyond. Happy coding!
Question & Answer Hub
Is Eclipse free to use?
Yep! Eclipse is open-source and free to download and use.
How much RAM does Eclipse need?
It depends on your project size and the plugins you use, but generally, 4GB is a good starting point. More RAM is always better for larger projects.
What’s the best way to learn Eclipse?
Hands-on practice is key! Start with a simple project, follow tutorials, and don’t be afraid to experiment. The Eclipse community is also super helpful, so don’t hesitate to search for answers online.
Can I use Eclipse on a Mac?
Totally! Eclipse runs on Windows, macOS, and Linux.
How do I uninstall Eclipse?
Just delete the Eclipse folder from your computer. No special uninstaller needed.